>[info] Redis分布式锁
**为什么需要分布式锁:**
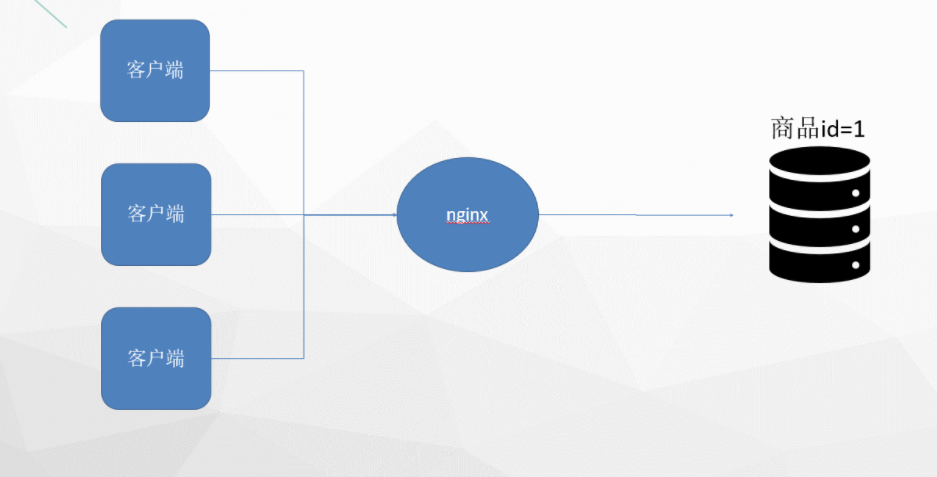
**保证互斥:**
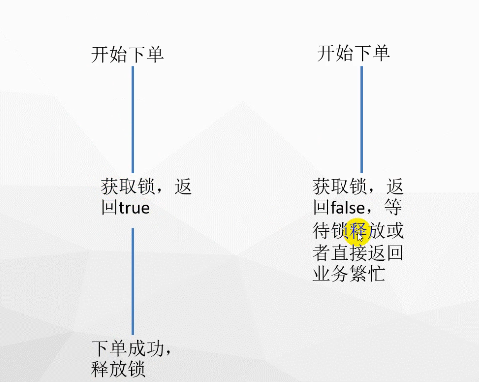
**死锁:**
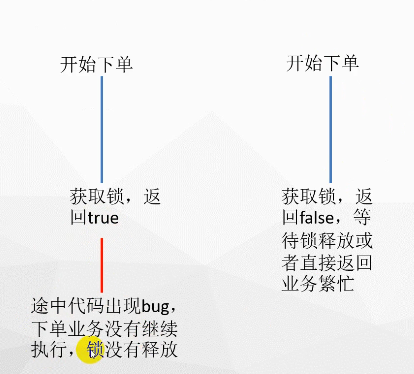
**加锁:**
~~~php
<?php
namespace App\Services;
use Illuminate\Support\Facades\Redis;
class RedisLockService
{
const LOCK_SUCCESS = 'OK';
const IF_NOT_EXISTS = 'NX';
const MILLISECOND_EXPIRE_TIME = 'EX';
const EXPIRE_TIME = 50; // millisecond => 50s
/**
* @param $key
* 加锁
*/
public function lock($key,$uuid,$expire_time = '')
{
if (empty($expire_time)){
$expire_time = self::EXPIRE_TIME;
}
$ret = Redis::set($key,$uuid,self::MILLISECOND_EXPIRE_TIME , $expire_time,self::IF_NOT_EXISTS);
if ($ret == self::LOCK_SUCCESS){
return true;
}else{
return false;
}
}
/**
* 释放锁
*/
public function unlock($key,$uuid)
{
$lua =<<<LUA_SCRIPT
if redis.call("get",KEYS[1]) == ARGV[1] then
return redis.call("del",KEYS[1])
else
return 0
end
LUA_SCRIPT;
$restful = Redis::eval($lua,1,$key,$uuid);
return $restful;
}
}
?>
~~~
**下单:**
~~~php
<?php
public function DistributedLock($id)
{
$RedisLock = new RedisLockService();
$key = "product_".$id;
$uuid = Uuid::uuid1($id);
$uuid = $uuid->getHex();
$return = "";
try{
if (!$RedisLock->lock($key,$uuid)){
return "当前业务繁忙,请稍后";
}else{
$ret = DB::table("fm_products")->where("id",$id)->first();
if ($ret->store >0){
$ret = DB::table("fm_products")->where("id",$id)->decrement("store");
if ($ret){
$return = "下单成功";
}else{
$return = "下单失败";
}
}else{
$return = "库存不足";
}
$RedisLock->unlock($key,$uuid);
return $return;
}
}catch (\Exception $exception){
$RedisLock->unlock($key,$uuid);
return $exception->getMessage();
}
}
?>
~~~
>[info] 内存淘汰策略
**定期删除:** 指的是redis默认是每隔100ms就随机抽取一些设置了过期时间的key,检查其是否过期,如果过期就删除。
**惰性删除:** 获取某个key的时候,redis会检查一下 ,这个key如果设置了过期时间那么是否过期了?如果过期了此时就会删除,不会给你返回任何东西。
**其他淘汰策略:**
~~~
volatile-random:从已设置过期时间的数据集中任意选择数据淘汰。
allkeys-random:从数据集(server.db[i].dict)中任意选择数据淘汰
volatile-lru:从已设置过期时间的数据集中挑选最近最少使用的数据淘汰。
allkeys-lru:从数据集中挑选最近最少使用的数据淘汰
volatile-ttl:从已设置过期时间的数据集中挑选将要过期的数据淘汰。
no-enviction(驱逐):禁止驱逐数据,这也是默认策略
~~~
**lru淘汰策略:**
根据冷热数据排序,最热数据排在最上面,反之冷数据反正最底端。
*****
按照英文的直接原义就是Least Recently Used,最近最久未使用法,它是按照一个非常著名的计算机操作系统基础理论得来的:最近使用的页面数据会在未来一段时期内仍然被使用,已经很久没有使用的页面很有可能在未来较长的一段时间内仍然不会被使用。基于这个思想,会存在一种缓存淘汰机制,每次从内存中找到最久未使用的数据然后置换出来,从而存入新的数据!它的主要衡量指标是使用的时间,附加指标是使用的次数。在计算机中大量使用了这个机制,它的合理性在于优先筛选热点数据,所谓热点数据,就是最近最多使用的数据!因为,利用LRU我们可以解决很多实际开发中的问题,并且很符合业务场景。
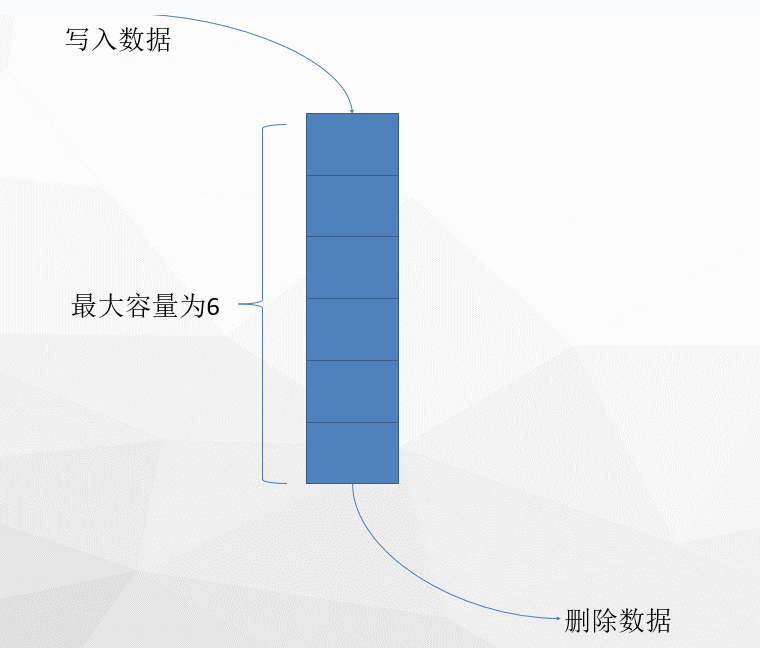
*****
**使用php实现lru策略:**
~~~php
<?php
namespace App\Services;
class LruService
{
/*
* 头部节点
*/
private $head;
/*
* 尾部节点
*/
private $tail;
/*
* 最大容量,大于淘汰部位节点指向上一个元素
*/
private $capacity;
/*
* 存储key对应的节点
*/
private $hashmap;
public function __construct($capacity)
{
$this->capacity = $capacity;
$this->hashmap = array();
$this->head = new LruNodeService(null,null);
$this->tail = new LruNodeService(null,null);
$this->head->setNext($this->tail);
$this->tail->setPrevious($this->head);
}
/**
* 获取元素
* @param $key
* @return null
*/
public function get($key)
{
if (!isset($this->hashmap[$key])) {
return null;
}
$node = $this->hashmap[$key];
if (count($this->hashmap) == 1) {
return $node->getData();
}
//先删除已经存在的结点
$this->detach($node);
//重新将新结点插入到头结点之后
$this->attach($this->head, $node);
return $node->getData();
}
/**
* 设置key value
* @param $key
* @param $data
* @return bool
*/
public function put($key, $data)
{
if ($this->capacity <= 0) {
return false;
}
if (isset($this->hashmap[$key]) && !empty($this->hashmap[$key])) {
$node = $this->hashmap[$key];
//重置结点到头结点之后
$this->detach($node);
$this->attach($this->head, $node);
$node->setData($data);
} else {
$node = new LruNodeService($key, $data);
$this->hashmap[$key] = $node;
//添加节点到头部节点之后
$this->attach($this->head, $node);
//检测容量是否达到最大值
if (count($this->hashmap) > $this->capacity) {
//如果达到最大值 删除尾节点左指针指向的元素
$nodeToRemove = $this->tail->getPrevious();
$this->detach($nodeToRemove);
unset($this->hashmap[$nodeToRemove->getKey()]);
}
}
return true;
}
/**
* 删除key
* @param $key
* @return bool
*/
public function remove($key)
{
if (!isset($this->hashmap[$key])) {
return false;
}
$nodeToRemove = $this->hashmap[$key];
$this->detach($nodeToRemove);
unset($this->hashmap[$nodeToRemove->getKey()]);
return true;
}
/**
* 添加新结点到头结点之后
* @param $head
* @param $node
*/
private function attach($head, $node)
{
//双向链表插入一个元素到头结点之后
$node->setPrevious($head);
$node->setNext($head->getNext());
$node->getNext()->setPrevious($node);
$node->getPrevious()->setNext($node);
}
/**
* 删除结点
* @param $node
*/
private function detach($node)
{
$node->getPrevious()->setNext($node->getNext());
$node->getNext()->setPrevious($node->getPrevious());
}
}
?>
~~~
~~~php
<?php
namespace App\Services;
class LruNodeService
{
private $key;
//key对应的内容
private $data;
//结点右指针
private $next;
//结点左指针
private $previous;
/**
* Node constructor.
* @param $key
* @param $data
*/
public function __construct($key, $data)
{
$this->key = $key;
$this->data = $data;
}
/**
* Sets a new value for the node data
* @param string the new content of the node
*/
public function setData($data)
{
$this->data = $data;
}
/**
* Sets a node as the next node
* @param Node $next the next node
*/
public function setNext($next)
{
$this->next = $next;
}
/**
* Sets a node as the previous node
* @param Node $previous the previous node
*/
public function setPrevious($previous)
{
$this->previous = $previous;
}
/**
* Returns the node key
* @return string the key of the node
*/
public function getKey()
{
return $this->key;
}
/**
* Returns the node data
* @return mixed the content of the node
*/
public function getData()
{
return $this->data;
}
/**
* Returns the next node
* @return Node the next node of the node
*/
public function getNext()
{
return $this->next;
}
/**
* Returns the previous node
* @return Node the previous node of the node
*/
public function getPrevious()
{
return $this->previous;
}
}
?>
~~~
>[info] 多级缓存
1. 提速
2. 减少服务器压力
3. 提高系统并发
*****
**Openresty安装:**
OpenResty由中国 人章亦春发起,提供了很多高质量的第三方模块。OpenResty 是一个强大的 Web 应用服务器,OpenResty可以快速构造出 足以胜任10K 乃至1000K以上并发连接响应的超高性能 Web 应用系统。360,UPYUN,阿里云,新浪,腾讯网,去哪儿网,酷狗音乐等都是 OpenResty 的深度 用户。
1. 安装依赖
~~~
yum install readline-devel pcre-devel openssl-devel
~~~
2. 获取安装包解压
~~~
wget https://openresty.org/download/ngx_openresty-1.9.7.1.tar.gz # 下载
tar xzvf ngx_openresty-1.9.7.1.tar.gz # 解压
~~~
3. 进入安装目录,编译安装
~~~
cd ngx_openresty-1.9.7.1/
./configure
make
~~~
*****
**使用Openresty-创建lua脚本:**
1. 存 redis
~~~
ngx.header.content_type="application/json;charset=utf8"
local cjson = require("cjson") --引入模块
local mysql = require("resty.mysql") --引入mysql模块
local uri_args = ngx.req.get_uri_args()
local product_id = uri_args['product_id']
local db = mysql:new() --初始化数据库
db:set_timeout(1000) --设置超时时间
local props = {
host = "192.168.29.108", --mysql ip地址
port = 3306, --mysql 端口
database = "starsky", --mysql 数据库
user = "starsky", password = "root" --用户名密码
}
local res = db:connect(props) --获得mysql连接
local select_sql = "select id,store from fm_products where id ='"..product_id.."'" --一条查询语句
res = db:query(select_sql) db:close() --执行
local redis = require("resty.redis") --引入redis
local red = redis:new() red:set_timeout(2000) --初始化 设置超时时间
local ip ="192.168.29.108"
local port = 6379
red:connect(ip,port)
red:auth("root")
red:set("product_"..product_id,cjson.encode(res)) --存储到redis
red:close()
ngx.say("{flag:true}")
~~~
2. 存本地
~~~
ngx.header.content_type="application/json;charset=utf8"
local uri_args = ngx.req.get_uri_args()
local product_id = uri_args['product_id']
local cache_ngx = ngx.shared.dis_cache;
local adCache = cache_ngx:get('productcache'..product_id);
if adCache == "" or adCache == nil then
local redis = require("resty.redis") --引入redis
local red = redis:new() red:set_timeout(2000) --初始化 设置超时时间
local ip ="192.168.29.108"
local port = 6379
red:connect(ip,port)
red:auth("root")
local rescontent=red:get("product_"..product_id)
ngx.say(rescontent)
red:close()
cache_ngx:set('productcache'..product_id, rescontent, 10*60);
else
ngx.say(adCache)
end
~~~
3. 配置 nginx
>[info] Redis - Key 的设计
1. 内存
2. 可读性
**主键存储查询:**
1. 表作为key的前缀
2. 列名作为key名
3. 主键值保证数据唯一
4. 数据列名
**例如:**
user:username:uid:1 starsky
product:uid:store 1
*****
**非主键存储查询:**
可以先根据列名设置一个key-value的值,然后在根据查询到的value去查询具体的数据,例如:
Set user:username:starsky:uid 1
$uid = Get user:username:starsky:uid
$user = get user: $uid:userinfo
- PHP
- ThinkPHP5
- 变量修饰符:input()
- 常用函数封装
- 01. 数据集转为树(Tree)
- 02. 生成订单号
- 03. 将时间戳转换为日期时间
- 04. 将字节转换为可读文本
- 05. 首字母头像
- 06. 随机小数/截取小数
- 07. 判断字符串是否序列化
- 08. 根据生日计算年龄
- 09. 判断是PC或WAP端
- 10. 请求网页返回code码状态
- 11. 计算代码执行时间
- 12. String 字符处理类
- 13. Http 请求类
- 14. StrRand 随机生成字符类
- 15. 获取当天开始时间和结束时间
- 16. 手机邮箱身份证
- 17. jsonEncode和jsonDecode助手函数
- 18. tp6跨域检测
- 19.日期转换为时间戳
- 20.excel导入日期格式问题
- 21.取整数函数常用的四种方法
- 22.PHPExcel导入导出
- 23.计算文件大小
- 24.计算按天数
- 25.文件夹不存在则创建
- 26.递归获取部门所有子级id
- 27.根据用户名生成默认文字头像
- 28.生成短信验证码
- 29.数据脱敏
- 30.根据身份证判断禁止未成年人下单
- 31.数组向指定位置插入元素
- 32.从字符串中获取城市名称
- 33.收货地址拆分省市区
- 34.根据时间戳获取本月开始-结束日期
- 35.循环每次查询指定数据集
- 36.判断日期是否符合范围
- Laravel6
- 01.Dingo API 2.0.0 扩展包
- 01.laravel 安装扩展包
- 02.创建端点
- 03.响应
- 04.api认证
- 监听sql 语句
- 安装easywechat
- 路由分组
- ThinkPHP6
- ThinkPHP6读写分离
- 定时计划任务
- 伪静态
- 多应用模式
- Join关联查询
- 跨域中间件
- extend扩展
- 1.操纵文件类
- 2.压缩包驱动
- Xdebug调试
- Easy Task开发文档
- 01.EasyTask介绍
- 02.EasyTask环境安装
- 03.EasyTask基础入门
- EasySwoole
- 999.状态类
- 01.EasySwoole快速开始
- 1.EasySwoole介绍
- 2.EasySwoole快速上手
- 3.EasySwoole基本管理命令
- 4.EasySwoole基础开始示例(CURD)
- 02.EasySwoole协程操作指南
- 1.什么是协程
- MySQL
- MySQL批量修改表前缀
- 重置【主键ID】
- 查看【文件存储位置】
- MySQL主从同步
- 添加用户权限
- MySQL主从复制集群
- 01.主从复制技术原理介绍
- 02.基于 binlog 主从复制搭建
- 03.基于binlog不影响业务搭建主从
- 04.主从复制 binlog 格式
- 05.主从复制过滤规则
- 06.在线增加从服务器
- 07.MySQL 双主复制
- 08.双主如何防止和解决主键冲突
- keepalived+haproxy+mysql双主高可用
- lvs+keepalived+双主mysql负载均衡
- MyCAT实现MySQL读写分离
- 09.多线程复制
- MHA
- 1.理解MHA高可用
- 2.搭建MySQL一主二从
- 3.建立节点互信
- 4.构建MHA
- 5.启动并测试MHA
- 6.修复宕机的Master
- 重置root密码
- MySQL 开发规范
- mycat
- 01.mycat 简介与安装
- 02.mycat 配置详解
- 03.mycat 切片规则
- 常用SQL语句大全
- 01.取数骚SQL
- 02.评估表数据体量SQL
- Linux
- Contos 7
- 常用命令
- 解压【zip】
- vmhgfs 挂载
- 跨主机免密码认证
- 宝塔Linux面板
- 01.宝塔专业/企业版一键脚本破解版
- Windows
- 服务器
- 调出【桌面图标】
- 查看【端口使用】
- 查看【操作日志】
- 查看【本地组策略】
- HTML
- URL编码参照表
- RabbitMQ
- 01.rabbitMQ 快速入门
- 1. 认识 RabbitMQ
- 4. 消费者生产者代码实例
- 3. 安装 amqp 扩展
- 2. RabbitMQ安装和启动
- 02.rabbitMQ 高级特性
- 1. 高级特性前言
- 2. 高级特性之一ack comfirm机制
- 3. 高级特性之二如何保证消息的100%接收(一)
- 4. 高级特性之二如何保证消息的100%接收(二)
- 5. 高级特性之三幂等性
- 6. 高级特性之四 return机制
- 7. 高级特性之五 限流机制
- 8. 高级特性之六 重回队列
- 9. 高级特性之七 TTL
- 10. 高级特性之八 死信队列
- 03.tp6简单应用 RabbitMQ
- 04.fanout 订阅/广播模式
- 05.topic 通配符模式
- Composer
- Composer 镜像
- Compsoer 基础使用
- Composer require 忽略依赖
- 微信公众号
- 返回错误代码说明
- 福利专区
- layuiAdmin框架模板
- Swoole
- 01.快速入门
- 1.swoole的概念介绍
- 2.安装swoole
- 3. 快速运用swoole与理解
- 02.快速上手swoole与网络协议
- 1.阻塞与非阻塞,同步与异步
- 2.HttpServer 加速php框架
- 3.WebSocket 简单运用
- 4.tcp 与 upd 与 定时器
- 5.理解进程-非详细
- 6.swoole结构
- 03.连接与tcp和upd
- 1.短链接与长连接
- 2.健康检查
- 3.tcp 和 udp对比
- 4.tcp粘包的问题
- 5.tcp粘包问题的处理
- 04.理解网络模型
- 1.linux内核与用户空间调度
- 2.网络io模型
- 3.构建worker结构iostar
- 4.理解stream系列函数
- 05.阻塞模型与非阻塞模型
- 1.构建基础worker结构
- 2.搭建工程的结构
- 3.实现基础版本的worker
- 4.完善worker模型
- 5.非阻塞模型与stream_select函数
- 6.补充
- 06.实现io复用与信号模型
- 07.实现异步io模型与Reactor模型
- 08.实现多进程reactor
- 09.详解task进程
- 10.swostar之http与websocket
- 11.think-swoole使用
- 12.swostar之构建基础核心结构
- 99.其他
- 西部数码
- 01.NginxSLB搭建
- 02.windows 服务器取消多登录
- PHP中高级面试题
- PHP基础面试题
- MySQL面试题
- Redis面试题
- es面试题
- rqbbitMQ面试题
- 魔鬼训练营
- 现场解答课-面试解答
- Laravel技术社区(干货题库汇总)
- 01.干货题库(一)
- 02.干货题库(二)
- 03.MySQL 常见面试题(1-25)
- 04.MySQL 常见面试题(26-58)
- Redis(2010期)
- 01.认识redis和安装
- 02.Redis数据类型与string类型与list类型
- 03.Redis数据类型之hash类型与zset类型
- 04.Redis数据类型之set类型与性能检测
- 05.Redis事务
- 06.Redis发布订阅与stream应用
- 07.Redis-lua脚本
- 08.缓存击穿,失效以及维度划分
- 09.缓存穿透,雪崩问题
- 10.Redis缓存问题以及分布式锁实现
- 11.redis持久化
- 1.redis-rdb持久化
- 2.redis-aof持久化
- 3.aof 和 rdb对比
- 12.redis主从复制
- 1.主从配置及原理
- 2.全量复制 和 部分复制
- 3.主从问题
- 13.redis哨兵机制
- 1.哨兵初识
- 2.redis哨兵原理
- 3.redis哨兵配置
- 4.redis哨兵问题
- 14.redis集群
- 1.集群搭建
- 2.故障转移
- 3.redis集群伸缩
- 999.redis 常用命令
- key
- string(字符串)
- hash(哈希)
- list(列表)
- set(集合)
- zset(有序集合)
- Stream
- Redis 发布订阅
- 事例
- Docker
- 1.docker快速入门
- 01.docker 了解
- 02.docker核心概念介绍
- 2.docker安装
- 3.docker基本使用
- 01.常用命令(镜像容器)
- 999.docker基本使用(示例)
- 01.docker部署es
- 02.docker部署lnmp
- 4.portainer可视化面板安装
- 5.docker镜像原理
- 01.docker镜像联合文件系统
- 02.docker分层理解
- 6.commit镜像
- 7.docker容器数据卷
- 01.容器数据卷基本使用
- 02.容器数据卷示例
- 03.容器数据卷具名挂载和匿名挂载
- 04.多个容器数据卷数据共享
- 8.dockerFile
- 01.Dockerfile介绍
- 02.Dockerfile指令说明
- 03.发布镜像到dockerhub
- 04.发布镜像到阿里云
- 999.Dockerfile示例
- 01.Dockerfile构建 centos 镜像
- 02.Dockerfile构建 tomcat 镜像
- 03.Dockerfile构建 redis 镜像
- 04.Dockerfile构建 lnmp 镜像
- 9.docker网络
- 01.理解docker0
- 02.容器互联-link
- 03.自定义网络
- 04.网络互通
- 999.redis集群部署实战
- 10.docker composer容器编排
- 01.docker-compose 介绍
- 02.安装docker-compose
- 03.docker-compose 基本命令
- 04.docker-compose yml 常用命令
- 999.docker-compose示例
- 01.构建 nginx 镜像(docker-compose)
- 02.构建 lnmp 镜像(docker-compose)
- 11.docker swarm集群
- 12.ci/cd之jenkins
- 01.jenkins介绍
- 02.jenkins安装(docker-compose)
- 13.k8s
- 01.什么是k8s
- 02.k8s使用场景
- 03.k8s部署架构分析
- 04.k8s运行架构分析
- Git
- 01.git理论和特点
- 02.gitlab安装配置以及项目创建
- 03.用户从windows接入并拉取+提交+修改代码提交
- 04.如何把团队弄进来一起开发
- 05.git基础操作,练习命令
- 06.如果你让三毛自己新建分支,并在上面开发这个时候三毛该怎么办呢?
- 07.在分支开发完毕之后,如何合并到master
- 08.2个分支合并,发生冲突的演示,以及如何解决?
- 09.为什么要用git工作流,git工作流的好处
- 999.git常用命令
- phpstudy
- localhsot不显示目录
- tp6获取不到header头Authorization问题(apache)
- 分布式架构
- elk日志系统
- 01.elk为何而生
- 02.标准的日志系统包括哪些
- 03.什么是elk
- 04.架构设计分析
- 05.elk实操部署
- elasticsearch(简称:es)
- 01.es的简介
- 02.es的安装前提与安装
- 03.es-head 与 kibana安装
- 04.es的概念
- 05.ik分词器
- 06.es对于restful的基本操作
- 07.es花式查询
- 07.01 基本使用增删改查
- 07.02 高级查询
- 07.00 es花式搜索示例
- 01.大型互联网架构演进过程
- 1.何为大型互联网架构
- 2.分布式架构的演变过程
- 02.分布式通信技术介绍
- 1.分布式亿级架构实战
- 2.分布式-集群-微服务
- 3.分布式通信
- 4.分布式通信协议
- 03.分布式通信-实战
- 1.分布式队列原理
- 2.分布式队列实战
- 3.RPC代码实战
- 04.主流分布式架构设计详解
- 1.分布式架构特性
- 2.CAP理论
- 3.BASE理论
- 05.consul服务注册发现
- 1.consul的工作原理
- 2.consul集群实战与操作
- 06.consul配置中心与实战
- 1.配置中心是个什么
- 2.consul实现配置中心
- 3.consul的基本实战操作
- 4.实战跨服务调用
- 07.分布式数据库
- 1.为什么要分库分表
- 2.动态伸缩
- 08.分布式实战数据库实战
- 09.分布式-分库分表实战
- 10.分布式事务
- 1.分布式事务
- 2.2PC-两阶段提交
- 3.3PC-三阶段提交
- 4.TCC
- 5.MQ事务最终一致性
- 11.分布式实战
- 12.elastic
- 13.elastic实战
- 14.elastic场景实战分析
- 15.elk
- 16.rabbitMQ
- 17.Kafka
- Redis、Nginx优化(2004期)
- 新能源汽车
- 数据表介绍
- 汽车租赁后台管理
- 微信小程序授权
- 微信小程序接口
- Go
- Beego 框架
- 命令行大全
- go_zero
- 01.基础部分
- 1.环境安装并输出HelloWorld
- 2.基础语法与数据类型
- 3.Go语言变量常量定义
- 4.Go语言作用域
- 5.Go常用占位符
- 6.Go异常处理
- 7.Go中的包
- 8.指针与fmt
- 9.下划线
- 10.数组(Array)
- 11.切片(Slice)
- 12.指针
- 13.Map
- 14.结构体
- 第三方库
- 01.strconv
- 02.json
- 03.air实时加载
- 04.gjson
- 05.lo
- 06.time
- 07.reflect(反射)
- 08.retry-go(重试机制)
- 09.strings
- 数据库操作
- 1.go操作mysql
- 2.go操作redis
- MongoDB
- 1.MongoDB 简介
- 2.MongoDB 安装
- 3.MongoDB 简单CURD
- 4.MongoDB 条件操作符
- 5.MongoDB Limit/Skip/Sort方法
- 6.MongoDB 索引
- 7.MongoDB 聚合与管道
- 8.MongoDB 复制(副本集)
- 99. 客户端安装与PHP操作
- Swoft
- 01.框架安装
- simpledingtalk修改点
- ThinkPHP6API基础模板
- uniapp
- 01.微信小程序获取页面路由参数
- 02.通用提示
- 03.缓存
- 04.日期转时间戳
- 05.VConsole调试
- Tailwind CSS
- 01.安装以及基础配置
- Vue
- 助手函数
- 1.时间戳格式化日期倒计时
- 2.获取不重复的id
- 3.获取正确的url路经
- 4.Object对象格式化为Query语法
- 5.数组转Tree
- 6.Tree转数组
- 7.判断值是否为空
- 9.数字前置补零
- 10.在线时间
- 11.html转义
- 12.pid形式数据转children形式
- 13.遍历children形式数据
- 14.全屏切换
- 15.获取屏幕宽高度
- 16.获取设备信息
- 17.百度高德地图坐标转换
- 18.深度克隆
- 19.获取变量类型
- 20.播放音频
- 21.导出excel
- 22.数字千分位
- 23.判断是否是外链
- 24.获取url参数
- ElementUI
- 1.表单验证
- 模板
- Yii2
- 01.SQL慢查询分块